Home
Welcome!
Metriport helps you access and manage your users’ and patients’ health and medical data, through an open-source universal API.
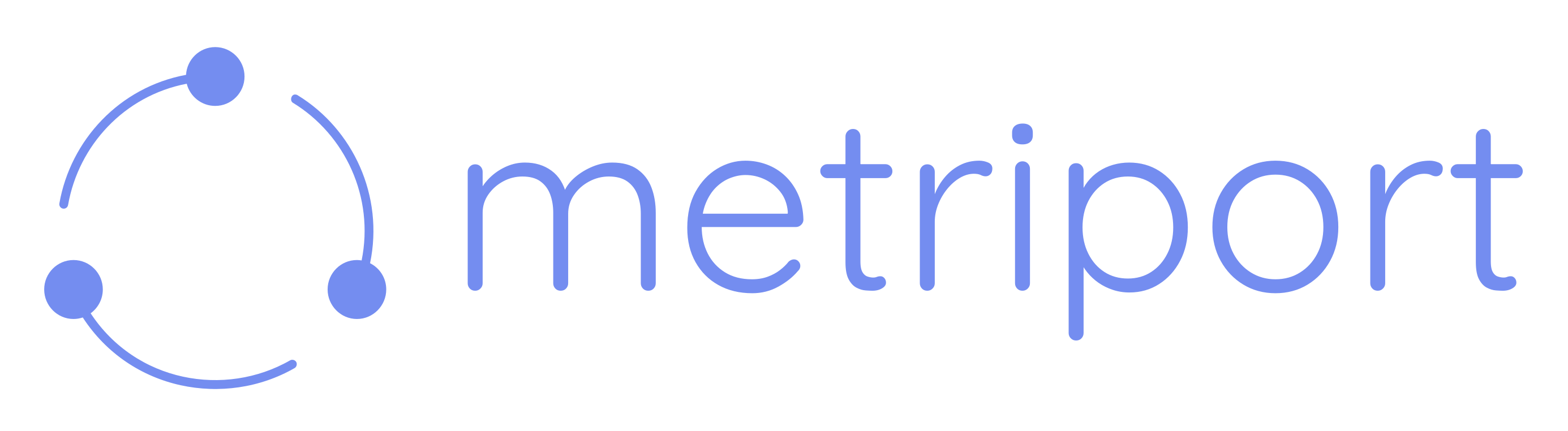
Medical API Quickstart
Follow this guide to get started accessing, managing, and exchanging your patients’ medical data.
Converter API Quickstart
Follow this guide to get started transforming your patients’ medical data to modern standards such as FHIR.
Source Code
Checkout our open-source GitHub repo and give us a star!
Contact
Any questions? Shoot us an email and we’ll get back to you quickly.